简介
- Spring Cloud是目前非常流行的微服务化解决方案,它将Spring Boot的便捷开发和Netflix OSS的丰富解决方案结合起来。如我们所知,Spring Cloud不同于Dubbo,使用的是基于HTTP(s)的Rest服务来构建整个服务体系。
- Eureka是Netflix开发的服务发现框架,SpringCloud将它集成在自己的子项目spring-cloud-netflix中,实现SpringCloud的服务发现功能。
服务端配置(Java)
- application.properties
1
2
3
4
5
6server.port=1111
eureka.instance.hostname=localhost
spring.application.name=localhost
eureka.client.register-with-eureka=true
eureka.client.fetch-registry=true
eureka.client.serviceUrl.defaultZone=http://${eureka.instance.hostname}:${server.port}/eureka/ - pom.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.didispace</groupId>
<artifactId>eureka-server</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
<name>eureka-server</name>
<description>Spring Cloud project</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.3.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka-server</artifactId>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Brixton.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project> - Application.java
1
2
3
4
5
6
7
8
9
10
11
12
13package com.didispace;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
public class Application {
public static void main(String[] args) {
new SpringApplicationBuilder(Application.class).web(true).run(args);
}
}
- application.properties
Node.js配置
安装eureka-client
1
npm install eureka-client --save
安装express
1
npm install express --save
注册服务
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24var Eureka = require('eureka-client').Eureka;
const client = new Eureka({
instance: {
app: 'PhoneQ',
hostName: 'localhost',
ipAddr: '127.0.0.1',
statusPageUrl: 'http://localhost:3333',
port: {
'$': 3333,
'@enabled': 'true',
},
vipAddress: 'test.something.com',
dataCenterInfo: {
'@class': 'com.netflix.appinfo.InstanceInfo$DefaultDataCenterInfo',
name: 'MyOwn',
},
},
eureka: {
serviceUrl: ['http://localhost:1111/eureka/apps/'],
},
});
client.start(function(error) {
console.log(error || 'Node server register completed');
});使用express挂起服务
1
2
3
4
5
6
7
8
9
10
11const express = require('express');
const app = express();
app.get('/health', (req, res) => {
res.json({
status: 'UP'
});
});
app.get('/', (req, res, next) => {
res.json({status: true,message:"It's works!"});
});
app.listen(3333);测试Eureka服务
启动Eureka服务,在浏览器输入http://localhost:1111/,看到类似下面的图片即说明服务成功开启:
使用Node.js注册
将上面Node.js注册服务部分代码保存到index.js,然后开启终端输入:node index.js,看到类似下面的结果说明服务成功注册:
查看注册服务
测试express服务
浏览器输入http://localhost:3333,可以看到服务已经挂起来了:
至此,Node.js成功引入Spring Cloud,看到这里的你也赶快动手试试吧,祝你成功!
使用Eureka将Node.js引入Spring Cloud
坚持原创技术分享,您的支持将鼓励我继续创作!
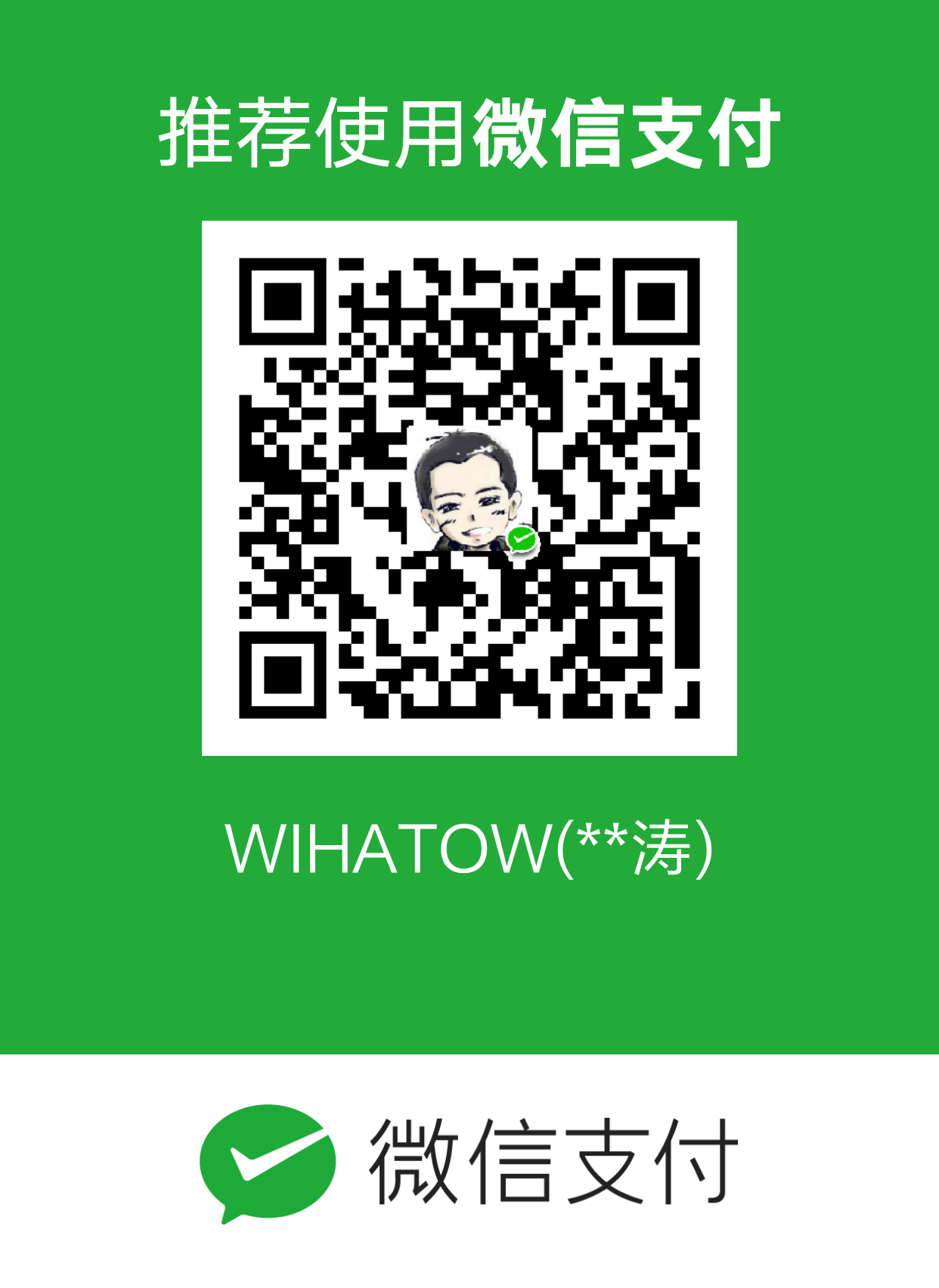
微信
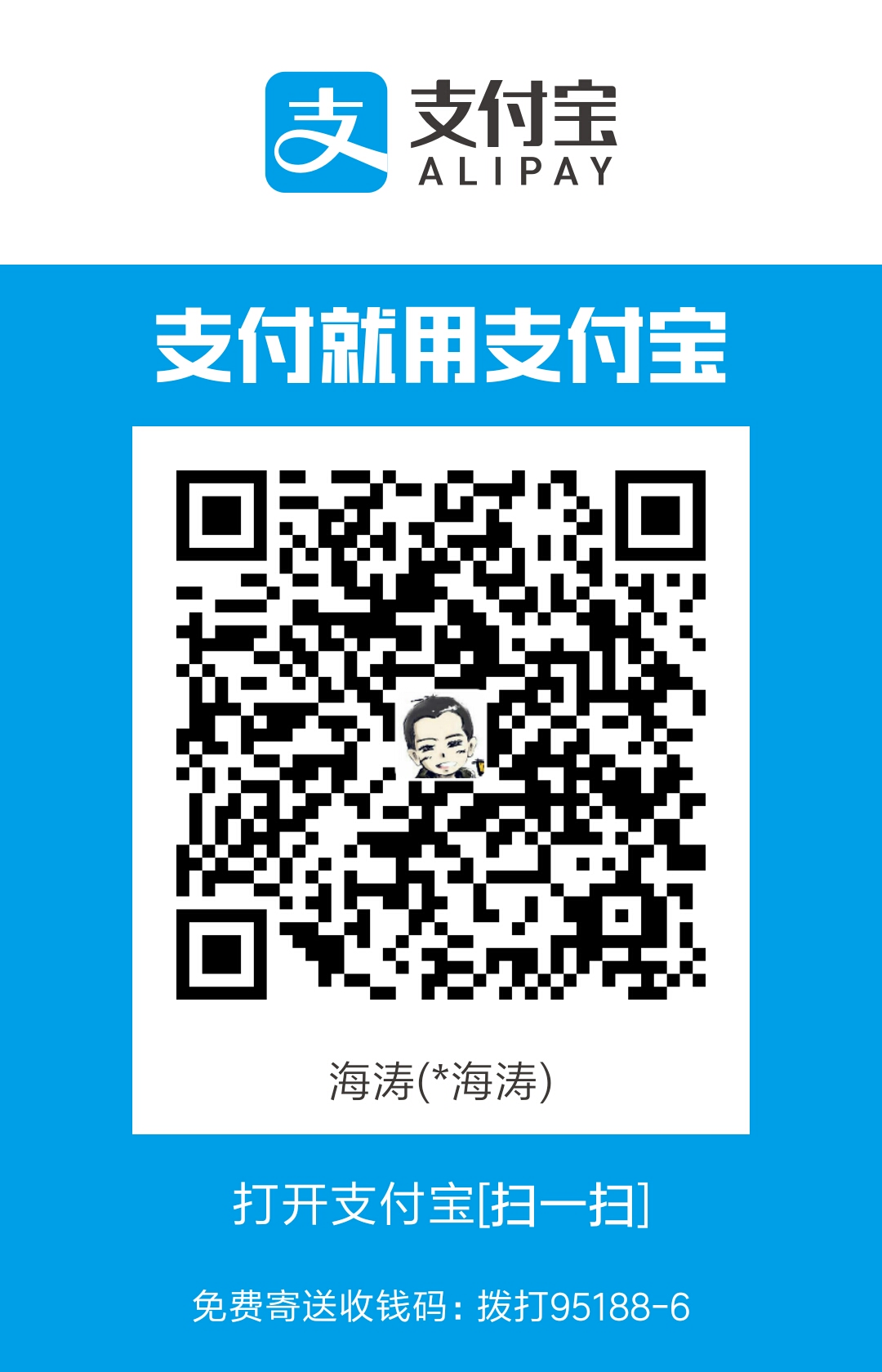
支付宝
- 本文链接: https://wihatow.github.io/2016/12/22/使用Eureka将Node.js引入Spring Cloud/
- 版权声明: 本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!